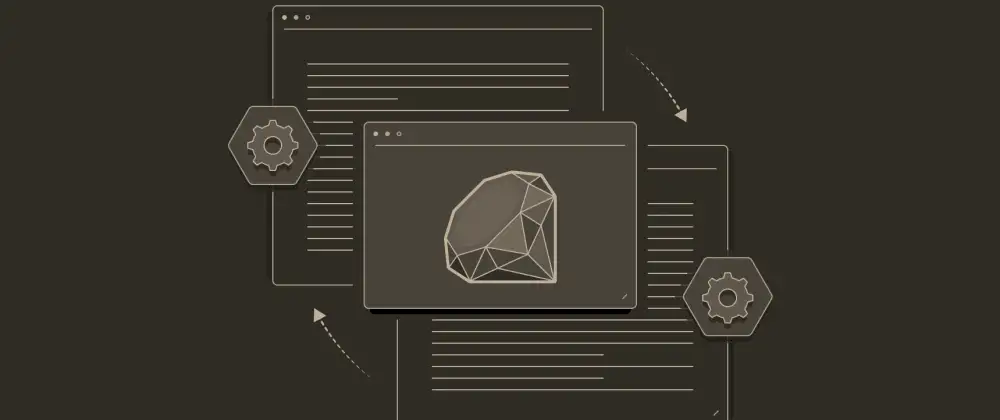
Ruby is a dynamic and flexible programming language that provides many features for metaprogramming. Metaprogramming is the ability to write code that generates or modifies other code at runtime, leading to more abstract, flexible, and reusable code. In this article, we’ll introduce you to the basics of Ruby metaprogramming and show you some simple examples to help you understand its power.
Ruby provides define_method for a dynamic method definition, class_eval for evaluating code within a class context, and instance_eval for evaluating code within an instance context. These features allow you to modify the behavior of classes and instances at runtime, leading to more flexible and easier-to-maintain code.
Here’s a simple example using define_method to create a set of methods that return the values of instance variables:
class Person attr_accessor :name, :age def initialize(name, age) @name = name @age = age end %w[name age].each do |m| define_method(m) do instance_variable_get("@#{m}") end end end person = Person.new("John", 30) puts person.name # outputs "John" puts person.age # outputs 30
In this example, we’re using define_method to define methods name and age that return the values of instance variables @name and @age, respectively. define_method takes a symbol representing the method name and a block defining the method's behavior. By using define_method to define methods dynamically, we can create flexible and reusable code that is easy to maintain.
And here’s an example using class_eval to define a method on a class:
class Person attr_accessor :name, :age end Person.class_eval do def greet puts "Hello, my name is #{name} and I'm #{age} years old." end end person = Person.new person.name = "John" person.age = 30 person.greet # outputs "Hello, my name is John and I'm 30 years old."
In this example, we’re using class_eval to define a method greet on the Person class. The method uses the name and age accessors to print a greeting message. class_eval takes a block of code and evaluates it within the context of the class, allowing you to modify the class at runtime.
Metaprogramming can be a powerful tool for writing more flexible and reusable code, but it can also make your code more complex and harder to understand. It’s important to use metaprogramming wisely and to understand its limitations. For example, while metaprogramming can simplify your code and make it more flexible, it can also make it harder to debug and maintain.
Additionally, it’s important to understand the core principles of object-oriented programming and the Ruby language itself, as this knowledge is crucial for effective metaprogramming. When you deeply understand the language and its underlying principles, you’ll be able to write more concise and maintainable code that leverages the full power of Ruby’s metaprogramming capabilities.
In conclusion, Ruby metaprogramming is a valuable tool for writing more flexible and reusable code. With the right understanding and experience, you can use its features to simplify your code and make it more readable, reusable, and flexible. It’s important to use metaprogramming wisely and to understand its limitations to write maintainable and efficient code. Practice and exploration are key to becoming a proficient and effective metaprogrammer in Ruby. As you become more comfortable with the concepts and techniques of metaprogramming, you’ll find that you can use it to create powerful and dynamic code that can be easily maintained and adapted to new requirements.
I hope this article has helped you understand Ruby's metaprogramming well. If you have any questions or want to share your thoughts on this topic, feel free to comment below. I would love to hear from you!
You must be logged in to comment on this blog.