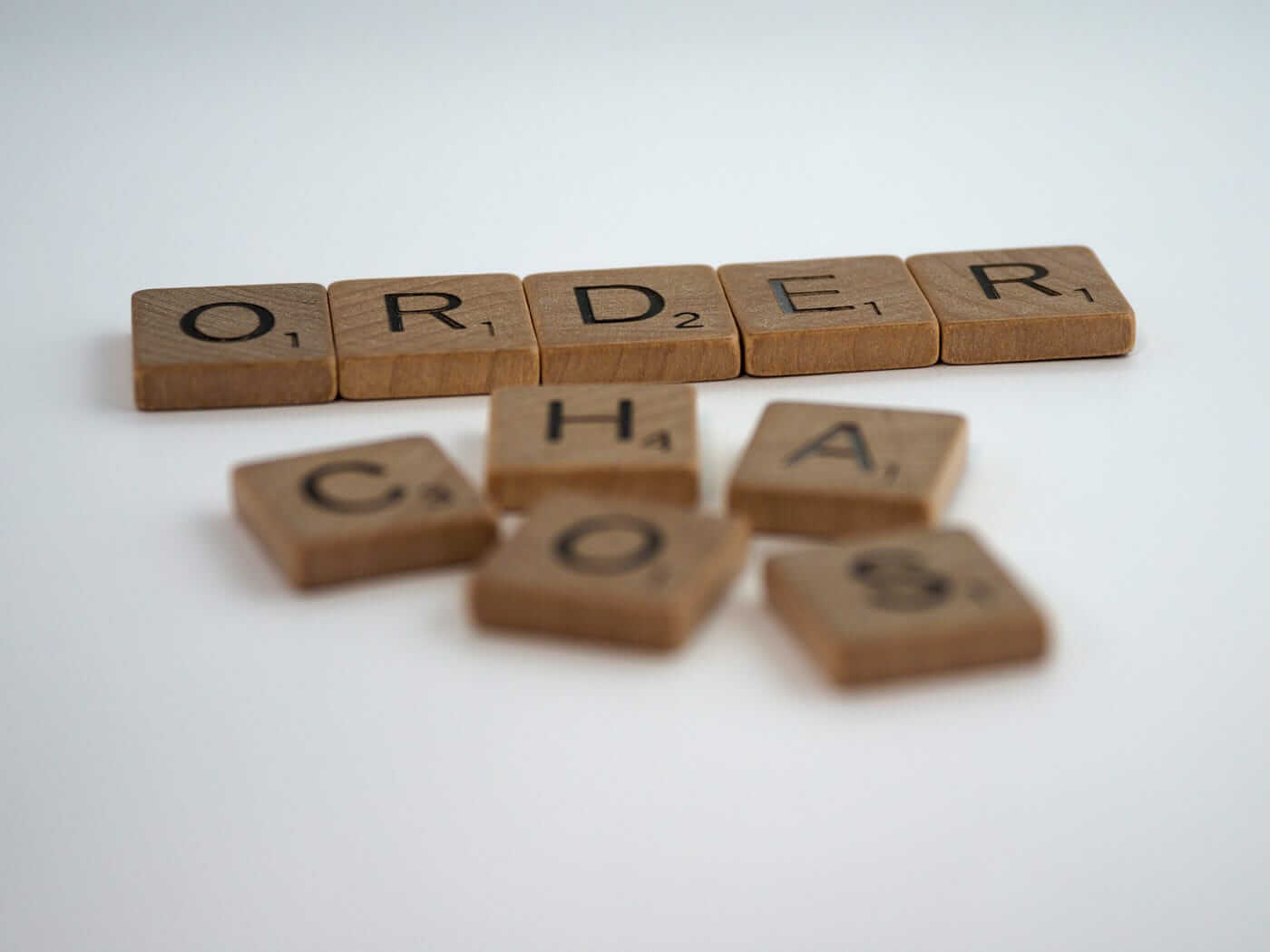
“Code as if the guy who ends up maintaining your code will be a violent psychopath who knows where you live”
John Woods
Introduction
Coding is an essential part of software development, and having readable, maintainable code is crucial for the long-term success of a project. The idea behind the above quote is to encourage developers to write code that is easy to understand and maintain. This blog will discuss some best practices for writing readable, maintainable code for beginner programmers.
As a programmer, you're likely familiar with the frustration of maintaining someone else's code. And while the quote above is a tongue-in-cheek way of reminding us to write our code in a way that's easy to understand and maintain, the sentiment behind it is profound.
No matter who ends up maintaining your code, be it a colleague, a future employer, or even yourself, the goal is to make it as easy as possible for them to understand and make changes to it. This is where self-documentation comes in.
What is Self-Documentation?
Self-documentation refers to writing code that is easy to understand without looking at external documentation or comments. This is achieved by using descriptive and meaningful variable names, writing clear and concise code, and using appropriate functions and method names that explain what the code does.
Why is Self-Documentation Important?
There are many reasons why self-documentation is essential, but here are a few of the most crucial:
- Saves Time: When the code is easy to understand, it saves the time that would have been spent trying to decipher what it does.
- Increases Collaboration: When code is well-documented, it makes it easier for multiple people to work on it and understand what's happening.
- Increases Maintainability: A well-documented code is easier to maintain, which means it's more likely to continue to work correctly over time.
How to Write Self-Documenting Code in Ruby?
Here are a few tips for writing self-documenting code in Ruby:
- Use Descriptive and Meaningful Variable Names: Avoid using abbreviations or single-letter names for variables. Instead, use descriptive and meaningful names that explain what the variable is used for.
- Write Clear and Concise Code: Avoid writing complex or overly verbose code. Instead, write clear and concise code so it's easy to understand what it does.
- Use Appropriate Functions and Method Names: Use function and method names that explain the code's actions. This makes it easier for others to understand what the code does without looking at the implementation.
- Use Comments: Use comments to explain what your code does, especially for complex or abstract code.
Coding Styles
Coding styles are guidelines that help ensure that code is consistent, readable, and maintainable. There are many coding styles, but some of the most common ones include the Ruby Style Guide, the Google Python Style Guide, and the Airbnb JavaScript Style Guide. These guides provide recommendations on line length, indentation, and naming conventions. For example, the Ruby Style Guide recommends using snake_case for method and variable names and two spaces for indentation.
Line Length
Line length is an essential aspect of coding style. Long lines of code can make code difficult to read and cause code to wrap on smaller screens. The recommended line length varies depending on the coding style, but keeping lines of code to around 80 characters or less is recommended.
Breaking Down Long Functions
Functions too long can also make code difficult to understand and maintain. To make code more readable, it is a best practice to break down extended functions into smaller, more manageable chunks. This makes it easier to understand what the function does and makes it easier to maintain the code in the future.
Coding Examples
Now let's look at an example to see the difference between code that is difficult to read and code that is easy to read.
# Before: class Invoice attr_reader :amt, :tax, :disc, :status def initialize(amt, tax, disc) @amt = amt @tax = tax @disc = disc @status = :unpaid end def ttl ttl = amt + amt * tax / 100 ttl = ttl - ttl * disc / 100 ttl end def pay @status = :paid end end # After: class Invoice attr_reader :total_amount, :tax_percentage, :discount_percentage, :payment_status def initialize(total_amount, tax_percentage, discount_percentage) @total_amount = total_amount @tax_percentage = tax_percentage @discount_percentage = discount_percentage @payment_status = :unpaid end def calculate_total_with_tax_and_discount taxed_amount = total_amount + total_amount * tax_percentage / 100 discounted_amount = taxed_amount - taxed_amount * discount_percentage / 100 discounted_amount end def mark_as_paid @payment_status = :paid end end
In the after version, the class name Invoice remains the same, but the function names calculate_total_with_tax_and_discount and mark_as_paid are more descriptive and explain what the code does. The variables total_amount, tax_percentage, and discount_percentage are also more descriptive and clarify what they represent. Using the variables taxed_amount and discounted_amount to calculate the amount after tax and discount, respectively, makes the code more readable. The return value discounted_amount is also descriptive and clarifies what the function is returning.
Best Practices from Major Companies
Leading companies have recognized the importance of writing code that is easy to understand, maintain, and extend. To achieve this, these companies have established best practices and coding styles to ensure the quality of their code. Airbnb and Google are two examples of companies that have made it a priority to write readable and maintainable code.
Airbnb's coding guide places a strong emphasis on code readability. One of their critical best practices is to write code that is easy to understand, even for someone unfamiliar. This is achieved by using descriptive variables and function names and documenting the code thoroughly.
Google's coding standards provide guidelines for indentation, line length, naming conventions, and more to ensure that code is easy to understand, maintain, and extend. Google also recognizes the importance of readable and maintainable code. They recommend writing well-structured, well-documented code and following established coding styles.
Writing high-quality code is essential for the long-term success of any project. Code that is easy to understand and maintain makes it easier for future developers to work with and extend, reducing the risk of errors and ensuring the project's success. By following best practices and coding styles, developers can ensure that their code is high quality and that future maintainers can work with it efficiently.
Conclusion
In conclusion, writing readable and maintainable code is crucial for the long-term success of a project. Taking the time to write high-quality code can help ensure the success of a project and make the lives of future maintainers much easier. By following best practices from major companies such as Airbnb and Google, developers can ensure that their code is high-quality and easy to understand and maintain.
We hope this blog has provided valuable information about writing readable and maintainable code. If you found this blog helpful, please share it with others who may benefit from it. If you have any questions or want to learn more about this topic, please feel free to contact us. We would be happy to help you in any way we can.
You must be logged in to comment on this blog.